I've been trying to arrange four images side by side, with two on the top row and two on the bottom. I want to ensure they stay consistent across all browser sizes except for mobile. Here is what I have attempted so far:
#imageone{ position: absolute; top:0px; width: 50%; padding:0px; } #imagetwo{ position: absolute; width: 50%; left:50%; } #imagefour{ position: absolute; width:50%; top:1000px; } #imagethree{ position: absolute; width:50%; left: 50%; top:1200px; }
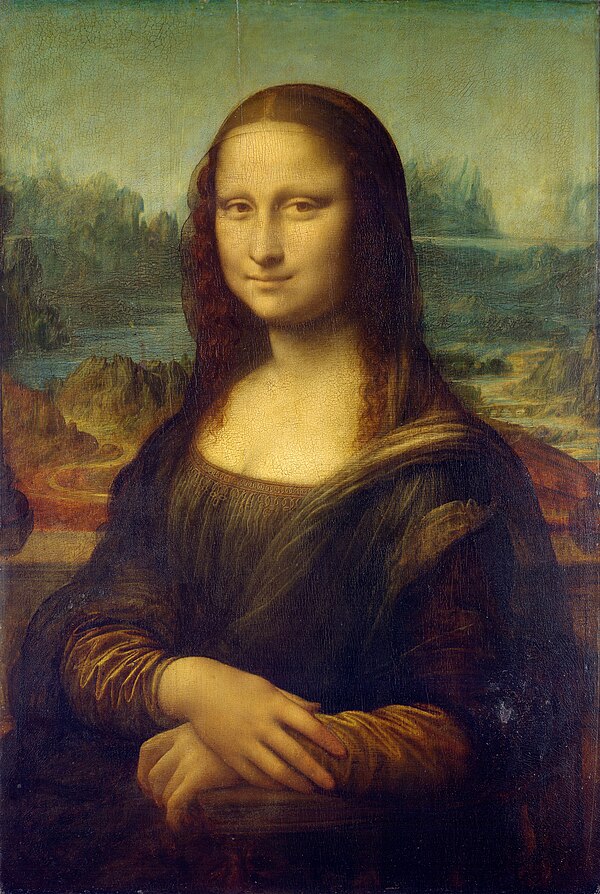
You can view it hosted on codepen here
Here is a visual description: link. Note: without padding, margin, or borderlines.